Crear un Web Api en .Net Core
Crea un archivo con la información requerida
Data.cs
using System.Collections.Generic;
using Trips.Data;
using System;
namespace Trips.Data
{
public static class Data
{
public static List<Trip> Trips => allTrips;
static List<Trip> allTrips = new List<Trip>()
{
new Trip()
{
Id=1,
Name="Vienna, Austria",
Description="Vienna, is one the most beautiful cities in Austria and in Europe as well. Other than the Operas for which it is well known, Vienna also has many beautiful parks...",
DateStarted = new DateTime(2017,01,20),
DateCompleted = null
},
new Trip()
{
Id=2,
Name="Carpinteria, CA, USA",
Description="Carpinteria is a city located on the central coast of California, just south of Santa Barbara. It has many beautiful beaches as well as a popular Avocado Festival which takes place every year in October...",
DateStarted = new DateTime(2019,02,22),
DateCompleted = new DateTime(2019,01,30)
},
new Trip()
{
Id=3,
Name="San Francisco, CA, USA",
Description="San Francisco is a metropolitan area located on the west coast of the United States. Some popular tourist features include the Golden Gate Bridge, Chinatown, and Fisherman's Wharf. There are a variety of popular food options...",
DateStarted = new DateTime(2019,01,27),
DateCompleted = new DateTime(2019,01,30)
},
new Trip()
{
Id=4,
Name="Tucson, AZ, USA",
Description="Tucson is a southwestern city in Arizona that is home to the University of Arizona. It was recently named a world gastronomy city, and is well known for its desert landscape and various bike races...",
DateStarted = new DateTime(2019,01,20),
DateCompleted = null
},
new Trip()
{
Id=5,
Name="Albuquerque, NM, USA",
Description="Albuquerque is a city located in New Mexico that is famous for its balloon festivals, picturesque views and references to TV shows.",
DateStarted = new DateTime(2015,01,20),
DateCompleted = new DateTime(2015,01,30)
},
new Trip()
{
Id=7,
Name="Munich, Germany",
Description="Munich is an important city in Germany, located in the heart of Bavaria. It's famous for its traditional Oktoberfest annual festival, and many nice lakes and parks...",
DateStarted = new DateTime(2019,01,20),
DateCompleted = null
}
};
}
}
Crear un modelo
Trip.cs
using System;
namespace Trips.Data
{
public class Trip
{
public int Id {get;set;}
public string Name {get;set;}
public string Description {get;set;}
public DateTime DateStarted {get;set;}
public DateTime? DateCompleted {get;set;}
}
}
Crear una interface
ITripService.cs
using System;
using System.Collections.Generic;
namespace Trips.Data
{
public interface ITripService{
List<Trip> getAllTrips();
Trip GetTripById(int tripID);
void UpdateTrip(int tripId, Trip trip);
void DeleteTrip(int tripId);
void AddTrip(Trip trip);
}
}
Crear una Servicio
TripService.cs
using System.Collections.Generic;
using System;
using System.Linq;
namespace Trips.Data
{
public class TripService : ITripService
{
public List<Trip> getAllTrips() => Data.Trips.ToList();
public Trip GetTripById(int tripID)
{
return Data.Trips.FirstOrDefault(n => n.Id == tripID);
}
public void UpdateTrip(int tripId, Trip trip)
{
var oldTrip = Data.Trips.FirstOrDefault(n => n.Id == tripId);
if (oldTrip != null)
{
oldTrip.Name = trip.Name;
oldTrip.DateCompleted = trip.DateCompleted;
oldTrip.DateStarted = trip.DateStarted;
oldTrip.Description = trip.Description;
}
}
public void DeleteTrip(int tripId)
{
var trip = Data.Trips.FirstOrDefault(n => n.Id == tripId);
if (trip != null)
{
Data.Trips.Remove(trip);
}
}
public void AddTrip(Trip trip)
{
Data.Trips.Add(trip);
}
}
}
Crear una Controlador
Trips.Controllers.cs
using Trips.Data;
using Microsoft.AspNetCore.Mvc;
namespace Trips.Controllers{
[Route("api/[controller]")]
public class TripsController: Controller{
private ITripService _service;
public TripsController(ITripService service)
{
this._service = service;
}
[HttpGet("SingleTrip/{id}")]
public IActionResult GetTripById(int id){
var trip = _service.GetTripById(id);
return Ok(trip);
}
[HttpGet("[action]")]
public IActionResult GetTrips(){
try
{
//throw new Exception();
var allTrips = _service.getAllTrips();
return Ok(allTrips);
}
catch (Exception ex)
{
return BadRequest("Error");
}
}
[HttpPost("AddTrip")]
public IActionResult AddTrip([FromBody]Trip trip){
if (trip != null){
_service.AddTrip(trip);
}
return Ok();
}
[HttpPut("UpdateTrip/{id}")]
public IActionResult UpdateTrip(int id, [FromBody]Trip trip)
{
_service.UpdateTrip(id, trip);
return Ok();
}
[HttpDelete("DeleteTrip/{id}")]
public IActionResult DeleteTrip(int id)
{
_service.DeleteTrip(id);
return Ok();
}
}
}
Modificar el archivo StartUp.cs
Agregar esta linea en el metodo ConfigureServices
services.AddTransient<ITripService,TripService>();
StartUp.cs
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.HttpsPolicy;
using Microsoft.AspNetCore.SpaServices.ReactDevelopmentServer;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Trips.Data;
namespace Trips
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
// In production, the React files will be served from this directory
services.AddSpaStaticFiles(configuration =>
{
configuration.RootPath = "ClientApp/build";
});
services.AddTransient<ITripService,TripService>();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseSpaStaticFiles();
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller}/{action=Index}/{id?}");
});
app.UseSpa(spa =>
{
spa.Options.SourcePath = "ClientApp";
if (env.IsDevelopment())
{
spa.UseReactDevelopmentServer(npmScript: "start");
}
});
}
}
}
Finalmente asi es como se veria la distribucion de los archivos en la solucion de Visual Studio
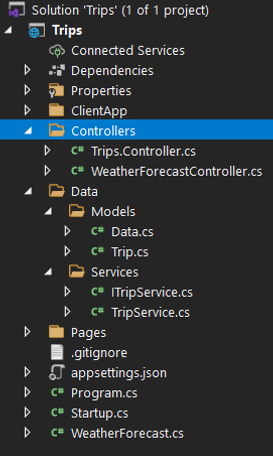
CREAR Y MODIFICAR UN ARCHIVO .TXT
MANEJO DE DIRECTORIOS Y ARCHIVOS
Comprimir y descomprimir archivos
Exportar tablas sql server a csv
Llenar LIST VIEW con tabla SQL SERVER
Crear una gráfica con tabla de SQL SERVER
Llamar un Web API MVC HttpClient
Llamar un Stored Procedure de SQL desde C#
Llamar un Stored Procedure de SQL desde C# para web api
Convertir JSON a Excel con Javascript
Convertir archivo JSON a listado objeto con C#
Servicio Get y POST en TypeScript y C#
Otros
Obtener divisas desde una página bancaria
Links the interes
Run dataset with SP and queries in C#
Llenar un DataSet al ejecutar un Stored Proc
C# Web API Sending Body Data in HTTP Post REST Client
Angular - HTTP POST Request Examples
Working with FormData in Angular 13
YouTube
Crear aplicación ANGULAR con API REST .NET 7 | ✅ Publicar en HOSTING✅