Crear una gráfica a partir de tabla SQL en C#
Código simple para crear una gráfica en C# a partir de la información almacenada en una tabla SQL SERVER.
A continuacion se muestran dos maneras de mostrar la tabla
using System;
using System.Data;
using System.Data.SqlClient;
using System.Windows.Forms.DataVisualization.Charting;
//Opción 1
public string LlenarGraficoTrafico(string id, Chart elgrafico)
{
try
{
SqlConnection con = new SqlConnection();
con = new SqlConnection("data source=" + ipServidor + ";uid =" + elUsuario + "; PWD=" + elpw + "; initial catalog= BD_GSM_NEW");
con.Open();
string textoComand = "SELECT * [dbo].[TRAFICO_3G] WHERE [Celula] ='" + id + "'";
DataTable dt = new DataTable();
SqlCommand cmd = new SqlCommand(textoComand, con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
da.Fill(dt);
string[] seriesArray = new string[5];
double[] pointsArray = new double[5];
elgrafico.ChartAreas[0].AxisY.Maximum = 70;
//llenamos las series columnas
for (byte i = 2; i < 7; i++)
{
seriesArray[i - 2] = dt.Columns[i].ToString();
}
foreach (DataRow renglon in dt.Rows)
{
for (byte i = 2; i < 7; i++)
{
pointsArray[i - 2] = Convert.ToDouble(renglon[i]);
}
break;
}
elgrafico.Series.Clear();
elgrafico.Titles.Clear();
// Se agrega un titulo al Grafico.
elgrafico.Titles.Add("Erlangs E");
// Agregar las Series al Grafico.
for (int i = 0; i < seriesArray.Length; i++)
{
// Aqui se agregan las series o Categorias.
Series series = elgrafico.Series.Add(seriesArray[i]);
// Aqui se agregan los Valores.
series.Points.Add(pointsArray[i]);
}
return "";
}
catch (Exception e)
{
return e.Message;
}
finally
{
con.Close();
}
}
//RESULTADO
//SEGUNDA OPCIÓN
public string LlenarGraficoTrafico2(string id, Chart elgrafico)
{
try
{
SqlConnection con = new SqlConnection();
con = new SqlConnection("data source=" + ipServidor + ";uid =" + elUsuario + "; PWD=" + elpw + "; initial catalog= BD_GSM_NEW");
con.Open();
string textoComand = "SELECT * [dbo].[TRAFICO_3G] WHERE [Celula] ='" + id + "'";
DataTable dt = new DataTable();
SqlCommand cmd = new SqlCommand(textoComand, con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
da.Fill(dt);
string[] encabezadosArray = new string[5];
double[] valoresArray = new double[5];
elgrafico.ChartAreas[0].AxisY.Maximum = 70;
//llenamos encabezados
for (byte i = 2; i < 7; i++)
{
encabezadosArray[i - 2] = dt.Columns[i].ToString();
}
//Llenamos la serie de los valores
foreach (DataRow renglon in dt.Rows)
{
for (byte i = 2; i < 7; i++)
{
valoresArray[i - 2] = Convert.ToDouble(renglon[i]);
}
break;
}
elgrafico.Series.Clear();
elgrafico.Titles.Clear();
//MANDAR LA INFORMACION A LA GRAFICA
Series series = elgrafico.Series.Add("TRAFICO");
for (byte i = 2; i < 7; i++)
{
series.Points.AddXY(encabezadosArray[i - 2], valoresArray[i - 2]);
}
return "";
}
catch (Exception e)
{
return e.Message;
}
finally
{
con.Close();
}
}
//Resultado
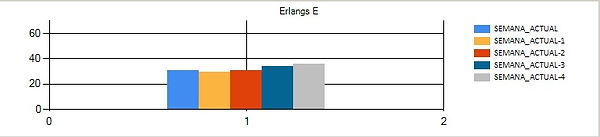
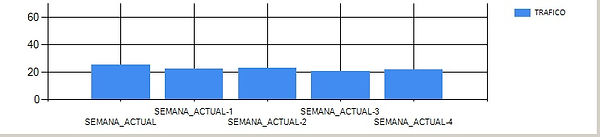
CREAR Y MODIFICAR UN ARCHIVO .TXT
MANEJO DE DIRECTORIOS Y ARCHIVOS
Comprimir y descomprimir archivos
Exportar tablas sql server a csv
Llenar LIST VIEW con tabla SQL SERVER
Crear una gráfica con tabla de SQL SERVER
Llamar un Web API MVC HttpClient
Llamar un Stored Procedure de SQL desde C#
Llamar un Stored Procedure de SQL desde C# para web api
Convertir JSON a Excel con Javascript
Convertir archivo JSON a listado objeto con C#
Servicio Get y POST en TypeScript y C#
Otros
Obtener divisas desde una página bancaria
Links the interes
Run dataset with SP and queries in C#
Llenar un DataSet al ejecutar un Stored Proc
C# Web API Sending Body Data in HTTP Post REST Client
Angular - HTTP POST Request Examples
Working with FormData in Angular 13
YouTube
Crear aplicación ANGULAR con API REST .NET 7 | ✅ Publicar en HOSTING✅